In This Topic
The Vertex Surface series can display arbitrary shapes and lines in 3D. With this surface type, you can specify the positions of each vertex as well as how the vertices are connected to form the surface mesh.
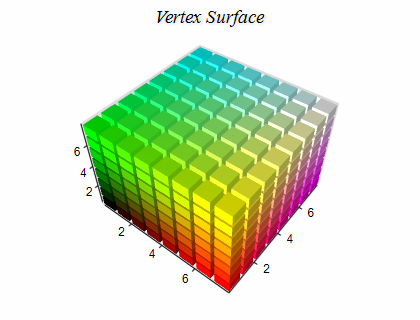
Creating a Vertex Surface Series
To create a grid surface you have to create an instance of the NVertexSurfaceSeries type and add it to the series collection of the chart. Surface series can be displayed only in 3D mode, so it is required to enable 3D for the chart hosting the series. The following code shows how to create a new vertex surface series and add it to the chart:
C# |
Copy Code
|
// setup chart
NCartesianChart chart = (NCartesianChart)chartView.Surface.Charts[0];
chart.Enable3D = true;
// set aspect 1:1:1
chart.ModelWidth = 50;
chart.ModelHeight = 50;
chart.ModelDepth = 50;
// set projection
chart.Projection.SetPredefinedProjection(ENPredefinedProjection.PerspectiveTilted);
// create a surface series
NVertexSurfaceSeries surface = new NVertexSurfaceSeries();
// add the series to the chart series collection
chart.Series.Add(surface);
|
Vertex Surface Data
The vertex surface data is a one-dimensional array of data points that consists of an XYZ value, and optionally a color value. The way this data is rendered on the GPU is controlled by the VertexPrimitive property of the surface, that accepts values from the ENVertexPrimitive enumeration:
ENVertexPrimitive |
Description |
Image |
Points |
Each vertex in the Data series is rendered as a point. |
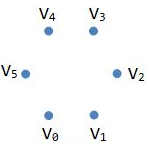 |
Lines |
Each pair of vertices in the Data series is rendered as a separate line segment. |
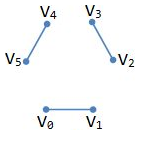 |
LineLoop |
The vertices in the Data series are rendered as a closed line. |
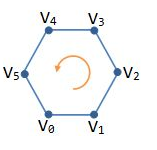 |
LineStrip |
The vertices in the Data series are rendered as a line. |
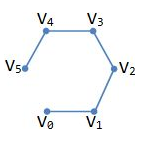 |
Triangles |
Each group of three consecutive vertices in the Data series is rendered as a triangle. For example vertices n, n + 1, and n+ 2 form a triangle, n + 3, n + 4, and n + 5 form a triangle and so on. |
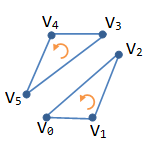 |
TriangleStrip |
Each tree adjacent points in the Data series form a triangle. For example n, n + 1, and n+ 2 form a triangle, n + 1, n + 2, and n + 3 form a triangle, and so on. |
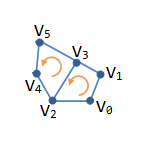 |
TriangleFan |
The first vertex participates in all triangles. Then each pair of adjacent vertices forms a triangle with the first vertex. For example 0, n, and n + 1 form a triangle, 0, n + 2, and n + 3 form a triangle and so on. |
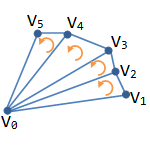 |
Quads |
Each group of four consecutive vertices in the Data series is rendered as a quad. For example n, n + 1, n + 2, and n + 3 form a quad, n + 4, n + 5, n + 6, and n + 7 form a quad and so on. |
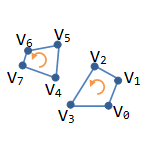 |
QuadStrip |
Each pair of vertices forms a quad with the next pair. For example n, n + 1, n + 2, and n + 3 form a quad, n + 2, n + 3, n + 4, and n + 5 form a quad and so on. |
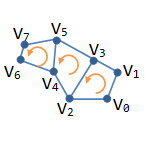 |
The following code adds three points to a vertex surface series and displays them as a triangle:
C# |
Copy Code
|
// obtain a reference to the Cartesian chart that is created by default
NCartesianChart chart = (NCartesianChart)chartView.Surface.Charts[0];
chart.Enable3D = true;
// set aspect 1:1:1
chart.ModelWidth = 50;
chart.ModelHeight = 50;
chart.ModelDepth = 50;
// set projection
chart.Projection.SetPredefinedProjection(ENPredefinedProjection.PerspectiveTilted);
// create a surface series
NVertexSurfaceSeries surface = new NVertexSurfaceSeries();
chart.Series.Add(surface);
surface.Name = "Vertex Surface";
// add some data
surface.VertexPrimitive = ENVertexPrimitive.Triangles;
// point 1
surface.Data.AddValue(3.0, 5.0, 1.4);
// point 2
surface.Data.AddValue(2.0, 1.9, 5.1);
// point 3
surface.Data.AddValue(1, 3.5, 4.7);
|
Vertex Surface With Custom Colors
When the grid surface FillMode is set to ENSurfaceFillMode.CustomColors or FrameColorMode is set to ENSurfaceFrameColorMode.CustomColors the surface will display the filling/frame with custom colors per vertex. In this case, you also need to pass a color value for each vertex. This is achieved by using the SetColor method of the vertex surface data object - for example:
C# |
Copy Code
|
surface.Data.UseColors = true;
surface.Data.SetColor(0, Color.Red);
|
Passing Large Amounts of Data
When you have to feed large amounts of data to a vertex surface series using the AddValue, SetValue, and SetColor methods will not be very efficient because it results in a function call for each value or color that is set. In such cases, you can consider adding data using unsafe code in which case you directly modify the surface data object in memory. The following code shows how to achieve this:
C# |
Copy Code
|
// create a surface series
NVertexSurfaceSeries vertexSurface = new NVertexSurfaceSeries();
// add the series to the chart series collection
chart.Series.Add(vertexSurface);
vertexSurface.Data.SetCount(1000);
unsafe
{
fixed (byte* pData = &vertexSurface.Data.Data[0])
{
Random random = new Random();
float* pValues = (float*)pData;
int itemSize = vertexSurface.Data.DataItemSize;
int count = vertexSurface.Data.Count;
// The order of the values is x, y, z. In this case we dynamically modify only x and z.
for (int i = 0; i < count; i++)
{
int dataPointOffset = i * itemSize;
pValues[dataPointOffset] = random.Next(1000);
pValues[dataPointOffset + 1] = random.Next(1000);
pValues[dataPointOffset + 2] = random.Next(1000);
}
vertexSurface.Data.OnDataChanged();
}
}
// notify the surface that data has changed
vertexSurface.Data.OnDataChanged();
|
See Also